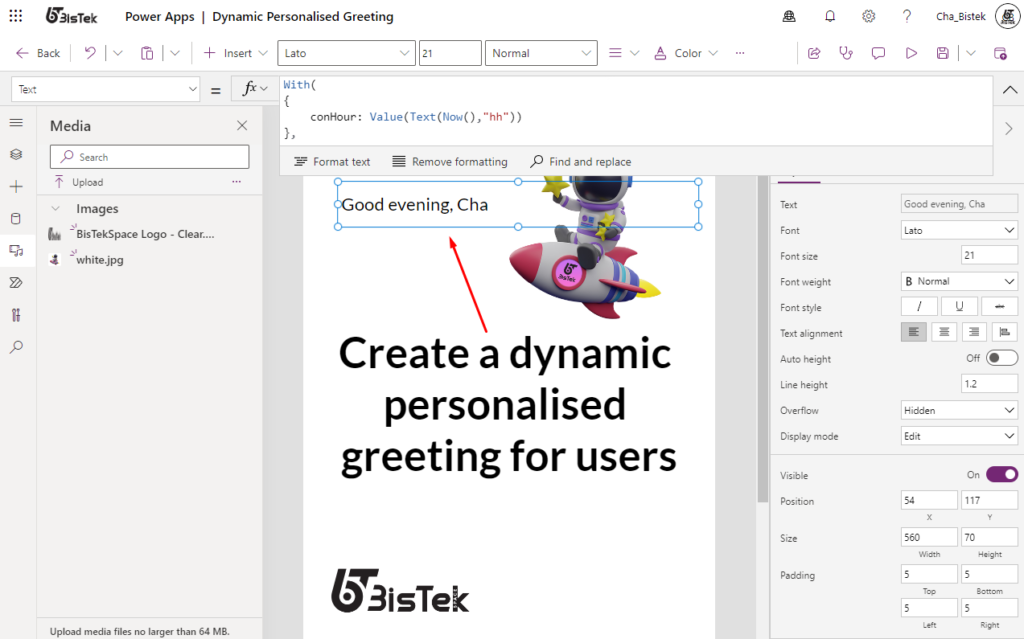
In this guide, you’ll learn how to implement a label that dynamically changes based on the time of day and incorporates the user’s first name for a personalised touch
//This is the code added to the label
With(
{
conHour: Value(Text(Now(),"hh"))
},
If(conHour>=0&&conHour<5,"Good night, ",
If(conHour>=5&&conHour<12,"Good morning, ",
If(conHour>=12&&conHour<18,"Good afternoon, ",
If(conHour>=18&&conHour<24,"Good evening, ",""))))
&
First(
ForAll(
Split(
User().FullName," "),
{Result:ThisRecord.Value}
)
).Result
)
C#Code explanation:
- The first part of the code is used to generate a greeting based on the current time. Here’s what it does:
- The
With
function is used to create a temporary variable calledconHour
, which is set to the current hour of the day using theNow()
function and theText()
function to format it as a two-digit number. - The
If
function is then used to check the value ofconHour
and generate a greeting based on the time of day:- If the hour is between 0 (midnight) and 5am, the greeting will be “Good night, “
- If the hour is between 5am and 12pm, the greeting will be “Good morning, “
- If the hour is between 12pm and 6pm, the greeting will be “Good afternoon, “
- If the hour is between 6pm and midnight, the greeting will be “Good evening, “
- If none of the above conditions are met, no greeting will be displayed (an empty string).
- The
&
operator is used to concatenate the greeting with the result of the second part of the code.
- The
- The second part of the code is used to extract the first name from the user’s full name. Here’s what it does:
- The
First
function is used to extract the first item from the result of theForAll
function. - The
ForAll
function is used to loop through each word in the user’s full name (which is obtained using theUser().FullName
function) and create a new record with a field calledResult
that contains the value of the current word. - The
Split
function is used to split the full name into an array of words based on the space character. - The
ThisRecord
function is used to refer to the current record being created by theForAll
function. - The
.Value
property is used to get the value of theResult
field for the current record.
- The
Overall, this code is used to generate a personalised greeting for the user based on the current time of day and their first name.